Cypress bursts into automated testing as an exciting alternative to well-known Selenium WebDriver. What is so unique in Cypress? Frontend developers created Cypress for frontend developers, but QA specialists can also take benefits from using it. This tool allows us to write JavaScript e2e tests, run them in the Chrome browser, and use Mocha test runner.
Why should you decide on API testing?
Working with data is what QA specialists do when it comes to writing API tests. It is one of the daily tasks for QA specialists working, e.g. in Scrum teams. API tests help in maintaining the functionality and logic of the application. Besides, developing modern applications requires that you combine graphical user interface tests with API tests so that e2e tests are delivered at these two levels.
But why is it so important to write API tests if at first glance it looks only like additional costs and efforts? First of all - bugs. The sooner the potential threats for the project are detected, the better. You can improve and automate this process using Postman, Selenium Web Driver or just with frameworks like Cypress. It has more advantages, but we covered this topic in other articles on API testing benefits on our blog.
REST API testing with Cypress
When it comes to using Cypress, many users think about front-end testing. But we can use Cypress when writing e2e API tests. If there is, e.g., REST API created in our application, automated API tests should be an integral part of the application's e2e tests. Cypress allows us to write these two types of tests. It is undoubtedly convenient because everything is done using one tool and one programming language - JavaScript.
In this article, we will work with the REST API - the REST acronym comes from the English words Representational State Transfer. It is a software architectural style in which we work with resources. The REST API from the example of this article is available here. In our test, we will get data about the most legendary pokemon - Pikachu. We are going to check if our GET method returns status code 200, whether the header contains 'application / json' and whether the name of the pokemon is Pikachu.
REST API testing - requirements
So let’s see what are we going to need to run our REST API test with Cypress. Make sure to:
Setting up the project
Ok, so now we are ready to set up the project. In the first step run the terminal, create a folder for API tests and enter it:
mkdir cypress-api-tests && cd cypress-api-tests
Create npm package:
npm init -y
Now install Cypress:
npm i cypress
You can open Cypress from your project root using command. npx is included with npm version > v5.2 but you can install it separately. We will see our test running interactively with the browser.
npx cypress open
It will start Cypress and create a cypress folder.
There are four subfolders in it: fixtures, plugins, support, and integration. In integration one there are some example tests that I have deleted. If you want to learn more about organizing tests, please take a look at Cypress docs. Additionally, in root, there is a cypress.json file created. Inside, you can see only curly brackets for now.
First API test
To run your first REST API test using Cypress, follow these steps:
- Go to cypress.json file and add the base URL that will be used in our test
{
"baseUrl": "https://pokeapi.co/api/v2/pokemon"
}
- Create “services” folder in the integration folder. Inside it create “getPokemonName.spec.js” file
- And then add this inside the file...:
describe('API Testing with Cypress', () => {
beforeEach(() => {
cy.request('25').as('pikachu');
});
it('Validate the header', () => {
cy.get('@pikachu')
.its('headers')
.its('content-type')
.should('include', 'application/json; charset=utf-8');
});
it('Validate the status code', () => {
cy.get('@pikachu')
.its('status')
.should('equal', 200);
});
it('Validate the pokemon\'s name', () => {
cy.get('@pikachu')
.its('body')
.should('include', { name: 'pikachu' });
});
});
...where we have created three tests:
- Should contain content-type: application/json; charset=utf-8,
- The status code should be 200,
- Should contain pokemon’s name - pikachu.
At the top, we need to import our baseURL that was created in the cypress.json file. Cypress has a built-in Mocha Test Runner - so there is no need to install it separately. Tests can be grouped inside describe groups. We write tests inside it function. We want to execute the request before each of the three tests in our describe group - for that we use beforeEach().
In our test, we use the Cypress request command to get pokemon information along with the GET method. Making requests is possible using a specific method, e.g., GET, POST, PATCH, or DELETE. In our test, we use the GET method, but there is no need to write it - if no method is defined, Cypress uses the GET method by default.
That’s why we can write:
cy.request('25')
Instead of:
cy.request('GET', '25')
In our test, we use also as() command because we want to assign an alias pikachu for later use.
In our file, we can group our tests with Mocha. Mocha additionally lets us running only one test by adding .only to it.
it.only('Validate the header', () => {
cy.get('@pikachu')
.its('headers')
.its('content-type')
.should('include', 'application/json; charset=utf-8');
});
Here one test only is being run:
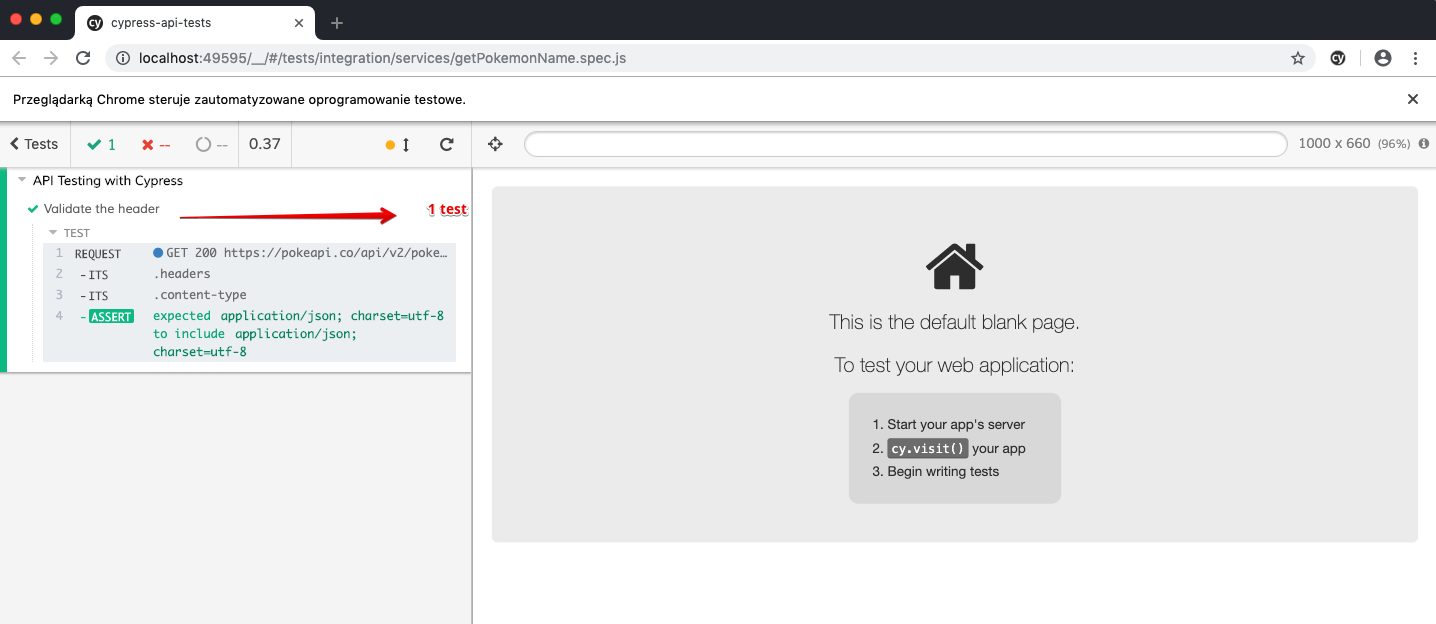
How to run our test? It was already said that we need to use npx cypress open command to run cypress To run all specs we should choose our test or click Run all specs button or just click on the one we want to run.
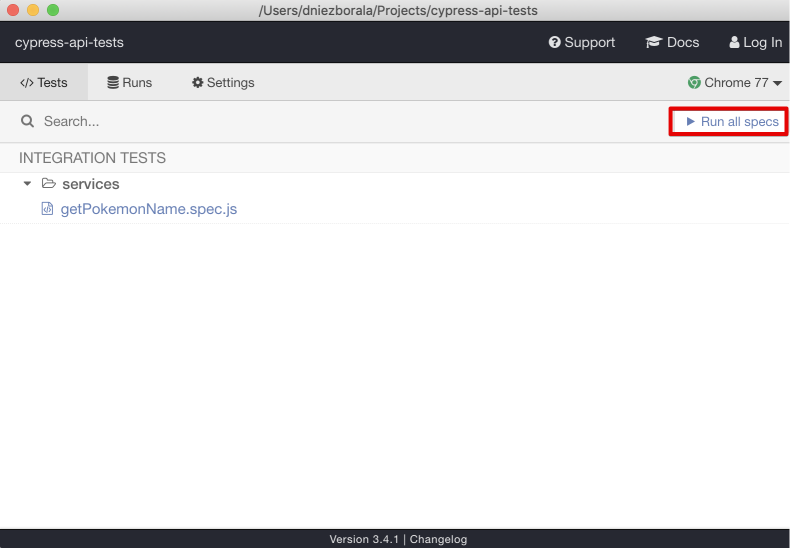
After that we got our test passed in the Cypress Test Runner:
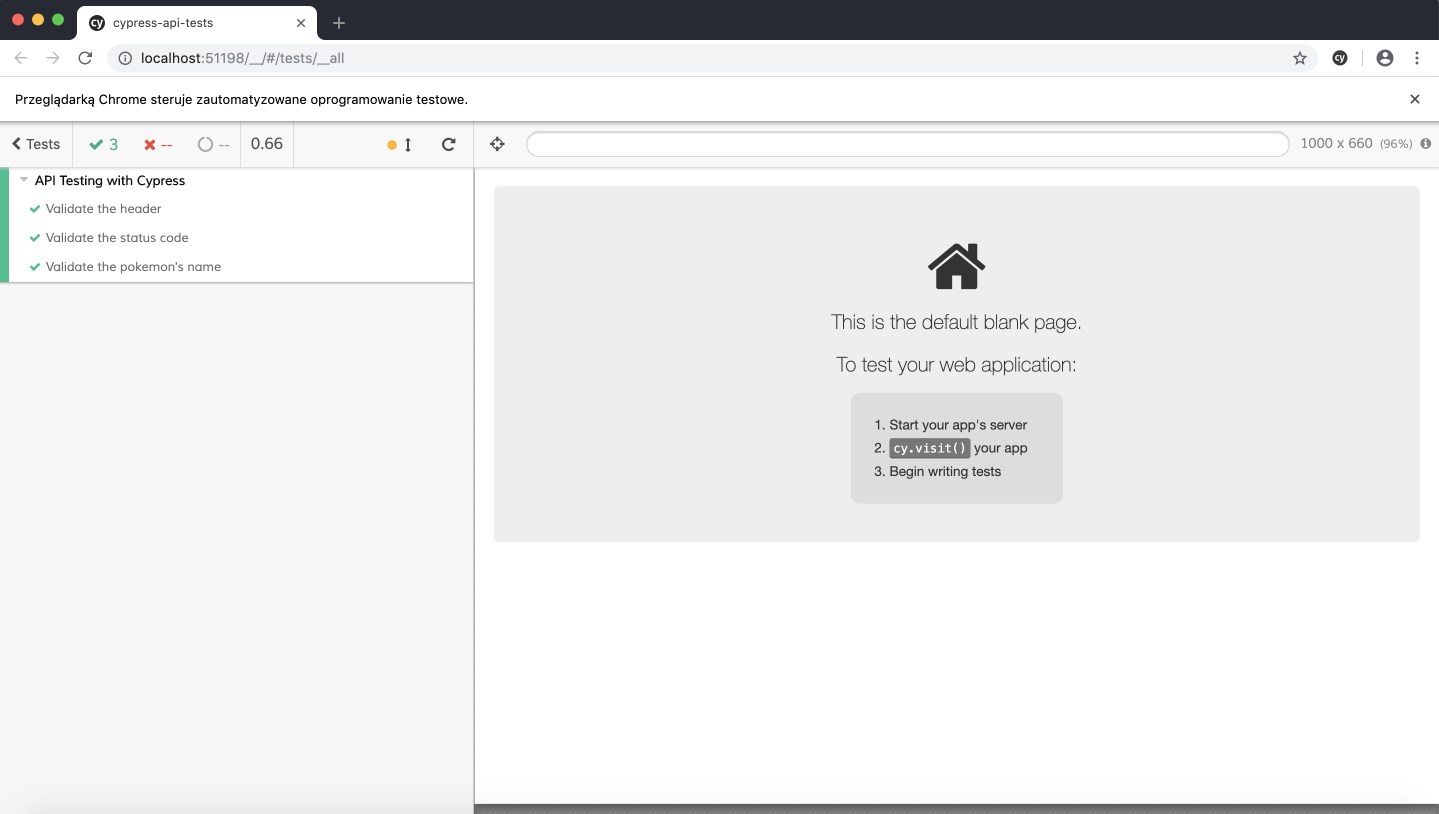
You can expand the results of the tests:
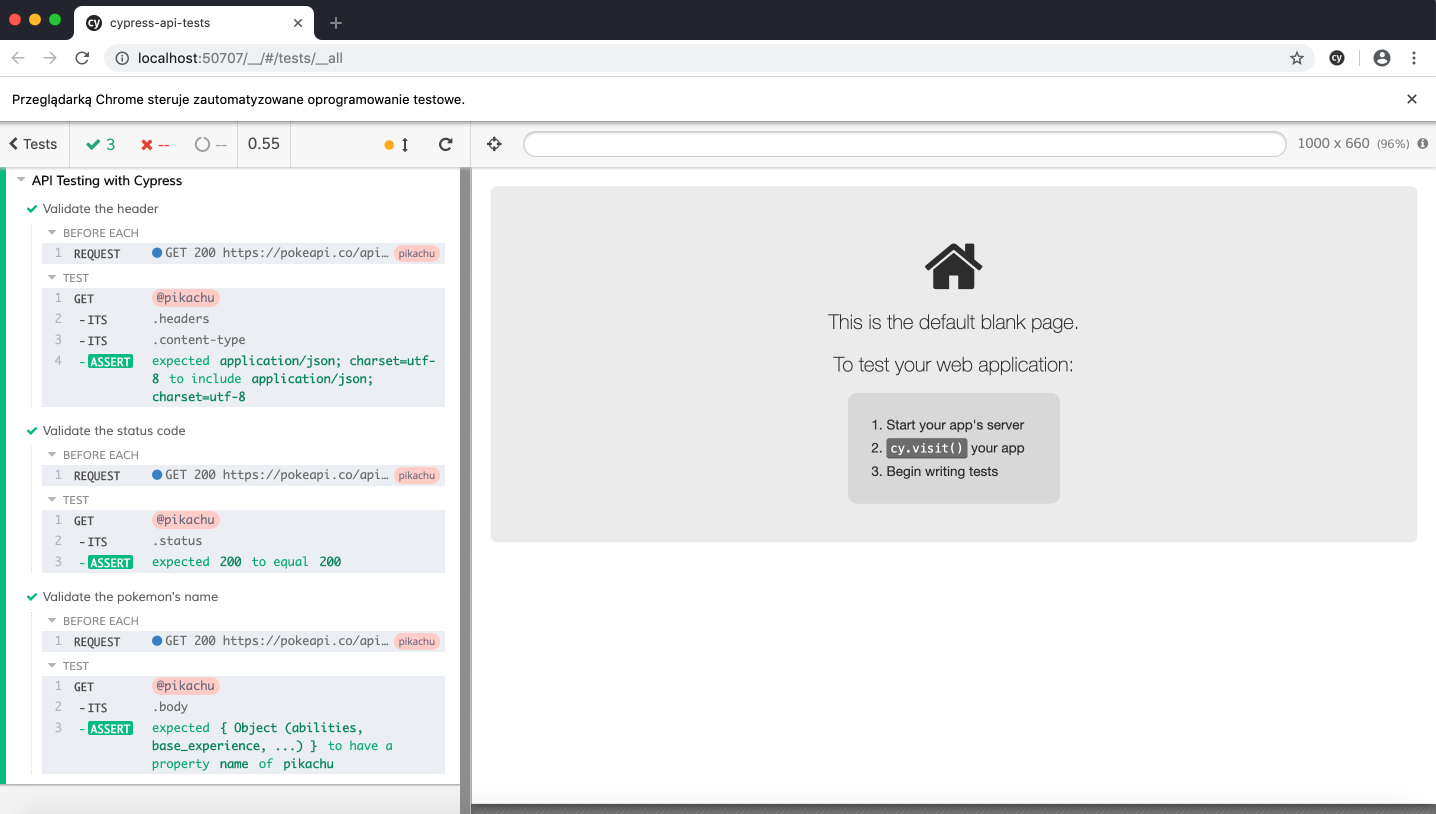
Is it worth to run tests with Cypress?
In summary, Cypress has a lot to offer to both frontend developers and QA engineers. It allows us to quickly and easily start working with test automation. Its unquestionable advantage is the possibility of using it in the project for both e2e UI and API tests, allowing us to work with JavaScript, Chrome, and Mocha as a test runner. It is an exciting alternative to Selenium WebDriver, and the support of the community centered around this framework is invaluable. Excellent documentation is another reason why you should give Cypress a chance and write your first test today.
Navigate the changing IT landscape
Some highlighted content that we want to draw attention to to link to our other resources. It usually contains a link .