Frontend world can be very intimidating for someone who’ve just decided to enter it. There is so much going on. You have HTML, CSS, Javascript, jQuery, then a never-ending list of fancy-named frameworks and libraries, like Angular, React, Vue, Ember, etc. It can be difficult especially for a total newbie which tries to learn frontend by himself to find his right spot. Been there, felt that. I strongly believe, and that’s an approach we praise in Merixstudio, that strong fundamentals are the key. Learn HTML5, CSS3 and JavaScript. Try to master them and when you’re done, you can try playing with frameworks. Don’t let yourself believe that Junior developer needs to know each one. Yeah sure, it is welcomed that you know what’s going on in this big world but better tread hard on the ground.
Let’s suppose you’ve already mastered basics and you want to get a little bit more educated. You're going through frameworks list and you see Vue.js. It looks promising. Let’s get deeper and find out more about this framework.
Vue.js - a perfect mix of React’s and Angular’s futures
According to Evan You, the creator of Vue.js, it’s a “progressive framework”, because it can be easily adapted to existing project and used only in some part of it. There is no requirement of building the whole project in this framework, like in case of React or Angular. Vue is focused on approachability and it has a shallow learning curve, which means that developers with basic knowledge of HTML, CSS, and Javascript can try it out. This framework is also presented as versatile, maintainable and testable. Developers with more experience in other frameworks can see some resemblance with those. For example, data binding and components are created similarly to those in React, however, template structure is more like in Angular. Vue’s author openly admits that he was picking the best parts of those frameworks to create something which will suit him in the most suitable way. “Sugar, spice, and everything nice, these were ingredients chosen...” to create Vue.js.
Small but powerful framework
This framework wasn’t prepared by any big company. Evan You created it from scratch supported by sponsorship from fundraised on Patreon. The whole framework has been rewritten twice from scratch as the creator wanted to make it as small and powerful as possible. Vue isn’t working only for small projects, big players like Laravel, Github or Alibaba are using it. What’s more, there is even suggested structure and naming convention for bigger projects shown in the Vue.js documentation.
The framework seems to be really small and lightweight compared to e.g. Angular, but it can be easily extended with additional features which work like adoptable pieces, e.g. routing or state management solution. They perform really nicely together since they’ve been all created by the same team. Vuex (Vue state management system) is designed particularly to cooperate with Vue. Vue.js also works really well with Redux, which I’ll want to describe more precisely in my next article. Above that all, Vue has amazing docs and guides, enriched with multiple examples and written in a really accessible way.
If you want to set up your Vue project, you can use the command-line tool which will guide you through the configuration process. Vue CLI 3.0 provides preconfigured set up on top of webpack 4.0 and it includes features like hot module replacement, tree-shaking, error overlays.
In the next paragraphs, I’d like to describe configuration and quick project set up for Vue.js.
Vue CLI for rapid development of a project
To use Vue CLI we must install a package via npm (npm install -g @vue/cli) or yarn (yarn global add @vue/cli). After installation, you can already use it to setup your project.
Use vue create your-project-name to create your project. After using this command you shall see:
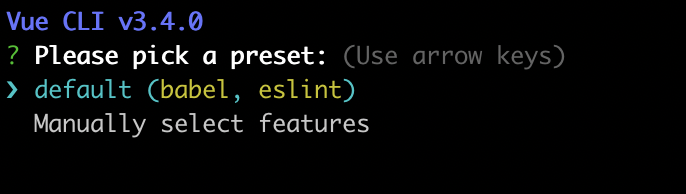
I’ve chosen manual set up and a list of all selectable features has been displayed:
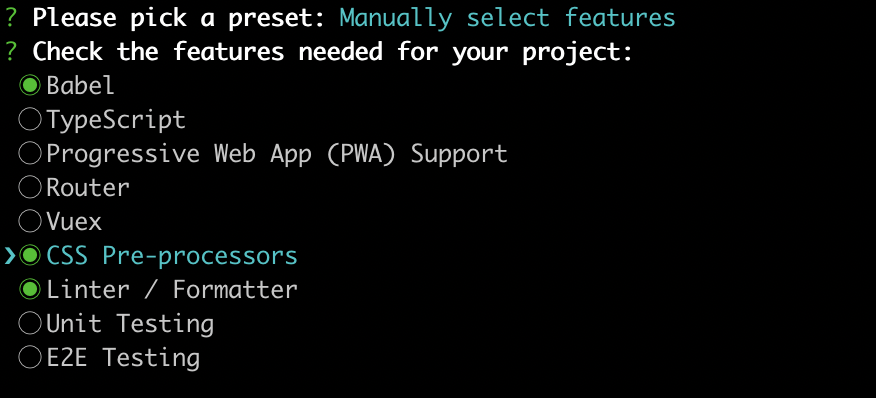
After that, you’ll see multiple steps to choose your project’s configuration. In the end, you should have something like this

When installation will be over, you’re ready to start your project with yarn serve command. An initial app should have corresponding files in its directory.
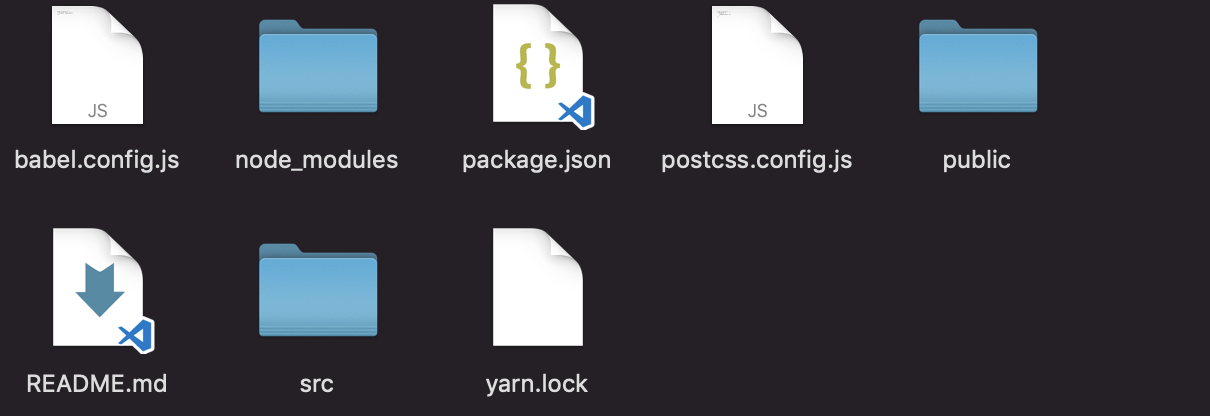
Vue syntax
As mentioned before, Vue.js has fantastic documentation but I’ll want to outline some of the basics of this framework on a simple app example.
If you want to use Vue in your existing project, you simply need to insert a script tag with vue source.
<script src="https://cdn.jsdelivr.net/npm/vue"></script>
However, if you’ve followed CLI configuration, you’ve already had some work done. Let’s go through it and discuss what’s it for.
Let's analyze main.js file - which is the project starting point created by the CLI tool that we used. This is where Vue instance is initiated. It’s done with new Vue({}).
import Vue from 'vue';
import App from './App.vue';
Vue.config.productionTip = false;
new Vue({
render: h => h(App),
}).$mount('#app');
Render method can be a little bit enigmatic at first but it’s shorthand from:
render: function (createElement) {
return createElement(App);
}
where “h” is taken from the term “hyperscript”. It is terminology used in multiple virtual dom implementations and means "script that generates HTML structures" because HTML is the acronym for "hyper-text markup language". So to sum up, in this place we render component “App” to our HTML which will be used as the main container for the whole project. The root component can be renamed to whatever you like but it’s good to keep it called “App”. You can find more about naming convention in Vue.js style guide.
Basic Vue.js component should have a name, template and optionally data. Please take a look at the example below:
Vue.component('like-button', {
data: function () {
return {
count: 0
}
},
template: '<button v-on:click="count++">Likes {{ count }} times.</button>'
});
A component can be also placed in one file with its script, styles, and template as in case of App.vue. Such component is called Single File Component in Vue.js and has .vue extension. It is highly recommended to use SFC while building bigger javascript application. Creating components this way helps to solve multiple problems, like global definitions (a unique name for each component), string templates (which can become poorly readable in case of more complex components), no CSS support or using HTML and ES5 JavaScript, instead of using preprocessors like Babel.
Single File Component is built with three sections: template, script, and styles. It can be easily imported and reused in any other component of the application. Below is an example of how our like button component will look like as Single File Component placed in a file called LikeButton.vue.
<template>
<button v-on:click="count++">Likes {{ count }} times.</button>
</template>
<script>
export default {
data: function () {
return {
count: 0
}
},
}
</script>
<style scoped>
button {
height: 40px;
font-size: 12px;
text-transform: uppercase;
}
</style>
You can see “scoped” in style tag which means that those styles will be applied only to the element of this particular Single File Component. A user can choose multiple ways to style his components (CSS preprocessor, e.g. Sass, Less, PostCSS) which can be specified while configuring the application with Vue CLI.
If you don’t like the idea of keeping everything in one file or you’re building big components which will have lots of styles and javascript applied you can divide them into separate files and import everything inside Single File Component.
<template>
<button v-on:click="count++">Likes {{ count }} times.</button>
</template>
<script src="./like-button.js></script>
<style src="./like-button.scss"></style>
As I mentioned Single File Component can be imported and used in any other component, e.g. in our App.vue component. A valid HTML tag for component will be always <name-of-component>, e.g. Like Button will have tag <like-button>. If you don't want to use default tag name, you can specify it in component’s declaration, e.g. ‘like-component’: LikeButton. In button tag, we can see basic data binding example which is text interpolation in double curly braces, also called “mustaches”. Thanks to v-on directive, after each click on a button, a count is increased by one and button text is automatically updated.
<template>
<like-button></like-button>
</template>
<script>
import LikeButton from '@/components/LikeButton;
export default {
components: {
LikeButton,
},
data: function () {
return {
count: 0
}
},
name: 'app'
}
</script>
While building scalable applications we deal with all kinds of data manipulation. Children components should get data from their parent components. We can quickly modify our LikeButton component and its parent App to suit this pattern.
<template>
<div id="app">
<img alt="Vue logo" src="./assets/logo.png" />
<like-button
v-on:count-clicks="count ++"
v-bind:count="count"
/>
</div>
</template>
<script>
import LikeButton from './components/LikeButton.vue';
export default {
name: 'app',
components: {
LikeButton,
},
data() {
return {
count: 0,
};
},
};
</script>
Now in App component we’re managing data and passing it to our Like Button component.
<template>
<button v-on:click="$emit('count-clicks')">Likes {{ count }} times.</button>
</template>
<script>
export default {
props: ['count'],
};
</script>
<style scoped>
button {
height: 40px;
color: #000;
font-size: 12px;
text-transform: uppercase;
}
</style>
As you can see count variable is now stored in the App component and it is modified in here. To pass count as property to child component we use v-bind directive. This directive will be used always while passing data. v-bind can be omitted and shortened, in our example, to :count="count". In child component property must be defined via props, e.g. props: ['count']. Vue has a few other directives, which are special attributes always used with v- prefix. In our example, we’ve chosen another one, v-on which is a perfect match when listening for DOM events, like clicking on the element. It is used twice - once on <like-button> tag as v-on:count-clicks="count ++" where we’re passing event handler count-clicks to children. The second time it is used in children component which on click action notifies parent via emit that it can fire count-clicks event. If you’re interested in more profound explanation and examples of their usage, please read more about Vue.js syntax.
Vue.js - challenge accepted?
In this article, we’ve got familiar with basic Vue.js concepts. It’s a very flexible, progressive framework which can be used for both complex and small applications as well as for some part of the existing project. We’ve taken a look at a very basic example with the configuration using Vue CLI. Thanks to this command line interface tool, a project can be easily set up even without specific knowledge of webpack. Thanks to its simplicity, Vue is good not only for more experienced developers but also for those which have basic knowledge of frontend programming. The framework has amazing documentation, is constantly improving and its community it’s thriving. Taking all this into consideration, it’s growing popularity isn’t a surprise.
Like what our developers do? Join our frontend team and raise your Vue.js skills. We’re looking for software developers - check our job offers!
Navigate the changing IT landscape
Some highlighted content that we want to draw attention to to link to our other resources. It usually contains a link .